An array is simply a series of instances of a particular variable type, given a single name and identified by that name plus an index number in brackets. Simple, huh?
You declare an array by specifying its type followed by an empty pair of brackets; and assigning it either a number of occurrences, or a set of values enclosed in braces and separated by commas. For example, here are some one-dimensional arrays.
String[] comics = {"Dick Tracy", "Prince Valiant"};
int daysInJanuary = 31;
int[] januaryTemps = new int[daysInJanuary];
(Notice that januaryTemps has to be initiated using the new keyword, even though it’s a primitive.) The items within an array are addressed by index numbers enclosed in brackets, where the first item is at index 0.
Notice, too, that the number of items in the array can be specified as either a variable or as a literal.
System.out.println(comics[0]);
causes “Dick Tracy” to be printed.
You can replace elements within the array like so:
comics[1] = "Doonesbury";
Arrays have a length() method that returns an int value which is the number of elements in the array–which is 1 greater than its highest index.
Arrays can have multiple dimensions, too, which are declared and used like this:
int[][] yearTemps = {{20, 30, 40}, {15, 25, 35}};
yearTemps[1][2] = 85;
Java also has the List interface (we’ll learn about that later), which manages collections of objects, but there are significant differences between arrays and Lists:
Array | List | |
Add or remove items? | No | Yes |
Can contain primitives? | Yes | No |
Can contain items of different types? | No | Yes* |
* You’d be foolish to store different classes of objects in a single List, but it can be done. Later we’ll discuss Java’s defense mechanism to combat such foolishness: Generics.
Iterating Over Arrays
There are two ways to iterate over arrays: the for-each method (which is simpler), and the index method.
// The for-each method
for (String comic : comics) {
System.out.println(comic);
}
for (int[] month : yearTemps) {
for (int day : month) {
System.out.println(day);
}
}
// The index method
for (int i = 0; i < comics.length; i++) {
System.out.println(comics[i]);
}
for (int i = 0; i < yearTemps.length; i++) {
for (int j = 0; j < yearTemps[i].length; j++) {
System.out.println(yearTemps[i][j]);
}
}
You’ve probably noticed that the main() method in every program you’ve run so far is passed an array of Strings. This array is the set of command line arguments. So your next exercise is to display them on the console.
Exercise
Write a Java class that displays the command line arguments on the console. You can do this from the main() method. Try this using both the iteration techniques above.
When you complete your coding, run the program once to establish a run configuration. Then use the Run/Run Configurations… menu command to open the Run Configuations dialog. Click on the Arguments tab where you can enter command line arguments like so:
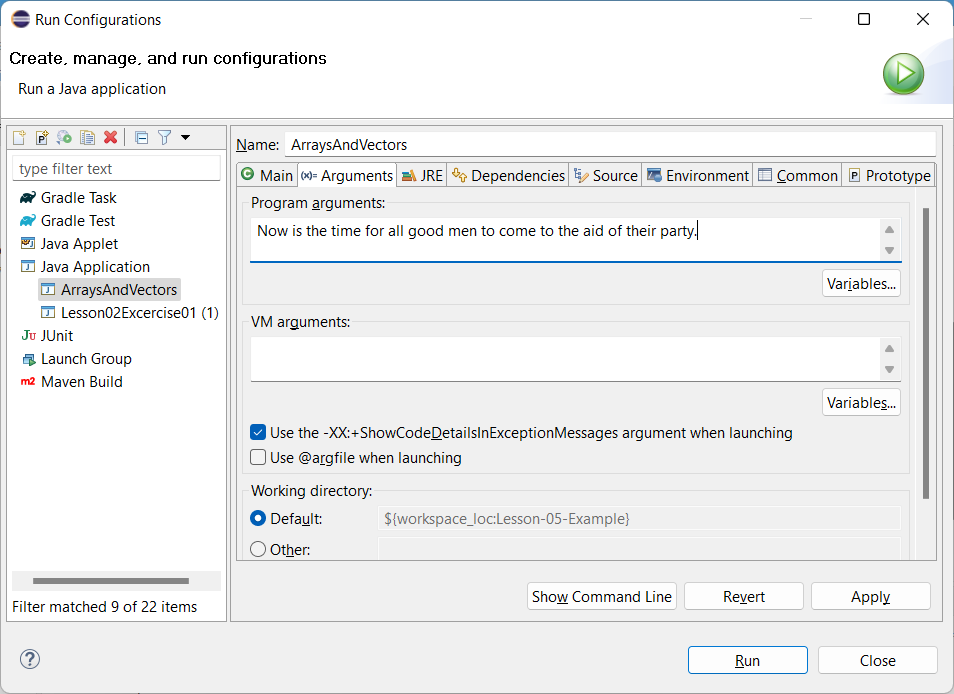
Click Run and your arguments should appear on the console:
Now
is
the
time
for
all
good
men
to
come
to
the
aid
of
their
party.
Stuck? Download a solution here and import it into your workspace.
The Arrays Class
Arrays are not objects. Nonetheless the Arrays class exists to provide static methods for manipulating arrays. (Didn’t we say a class is often just a collection of useful code?) These functions include:
- Converting an array to a List
- Sorting all or part of the array
- Conducting a binary search
- Copying all or part of the array to a new array of different length
- Comparing two arrays for equality
- Filling all or part of the array with a specific value
- Calculating a hash code from the array contents
For example:
int[] myArray = new int[250];
Arrays.sort(myArray);
Vectors
A Vector is essentially the same as a List. In fact, the Vector class has been deprecated, meaning it’s going to disappear from a future version of Java. A separate discussion isn’t worth the pixels. Forget I mentioned it. See the lessons on Collections and Lists instead.
Next: Enumerations