When we left off, we were looking at a poorly-formatted piece of source code chock-full of errors. It’s time to fix that.
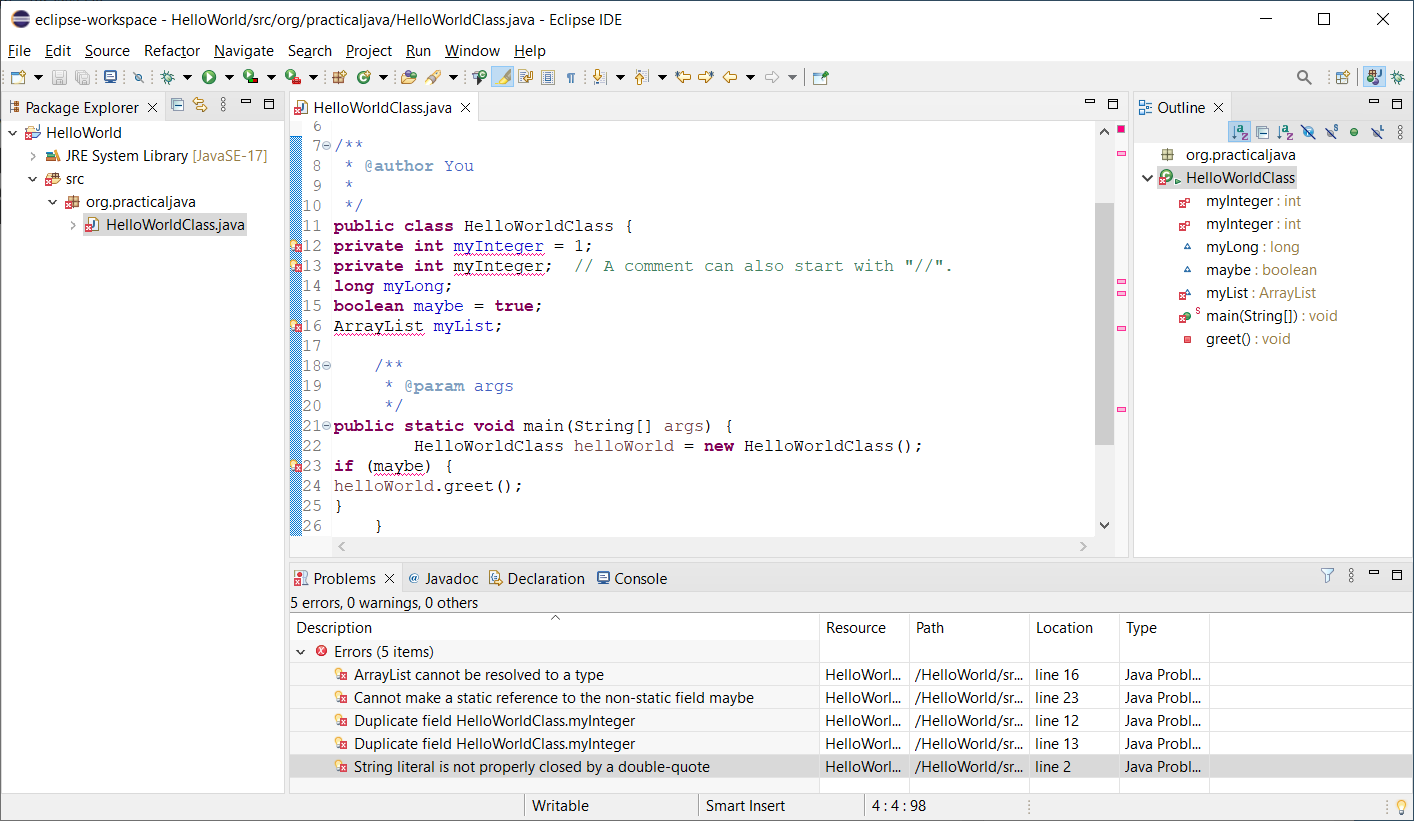
In Eclipse terminology, the layout you’re seeing is a perspective–specifically the Java perspective, which is used for working with Java programs. Each of the windows is a view: the Package Explorer view, the Problems view, the Console view, the Outline view, etc. You can add or remove views to or from the perspective, drag the views around the screen by their tabs, and resize them.
Let’s focus on the Problems view. We’ve got 5 errors. (There’s a red-circled X on each line with an error as well.) Let’s correct them.
Using “organize imports” to resolve types
Right-click on “ArrayList cannot be resolved to a type.” Press Ctrl+Shift+O, or select the menu item Source/Organize Imports, and a new line appears in the code window:
import java.util.ArrayList;
Organize imports adds needed import statements, removes unneeded ones, and sorts those that remain. It’s a nice way to clean up your code, so use the operation freely. In fact, I set an option in my Eclipse preferences (menu Window/Preferences/Java/Editor/Save Actions) to organize imports whenever I save.
Note the asterisk on the editor tab; this tells you that the source code has been modified but not saved. Press Ctrl+S (or File/Save) to save the file, and the error disappears (along with the asterisk).
Using Quick Fix
Now let’s address “Cannot make a static reference to the non-static field maybe.” (“maybe” is the name of something in the program, not an adverb.) Right-click on the error message and choose “Quick Fix” from the popup menu.
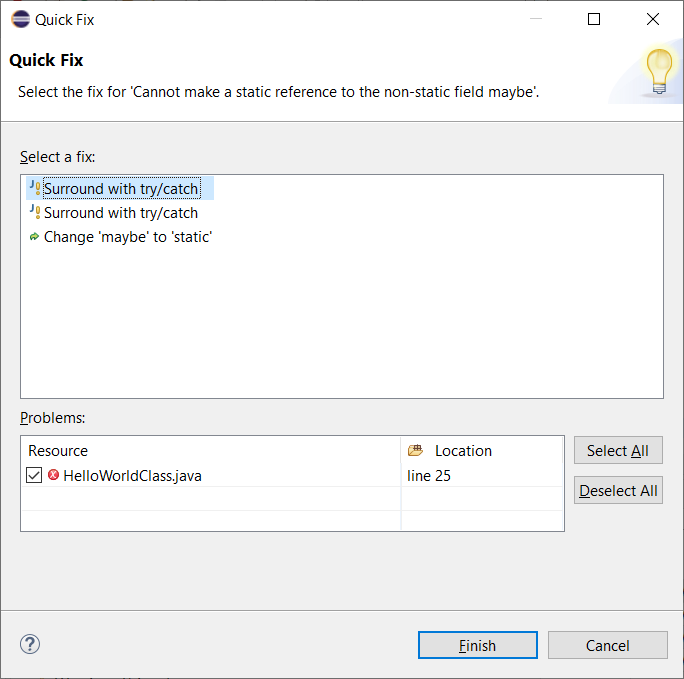
We need to change “maybe” to make it static–and yes, we’ll explain later what that means. Click on that option in the upper window and click Finish. Save the file again and poof! another error gone.
Resolving duplicate field names
Next is “Duplicate field HelloWorldClass.myInteger.” Just remove one or the other of the lines starting “private int myInteger”.
Now double-click on the error message, “String literal is not properly closed by a double-quote. The editor will take you to the line containing the error, which we’ll resolve by just deleting the line altogether. Save the file and we’re left with this:
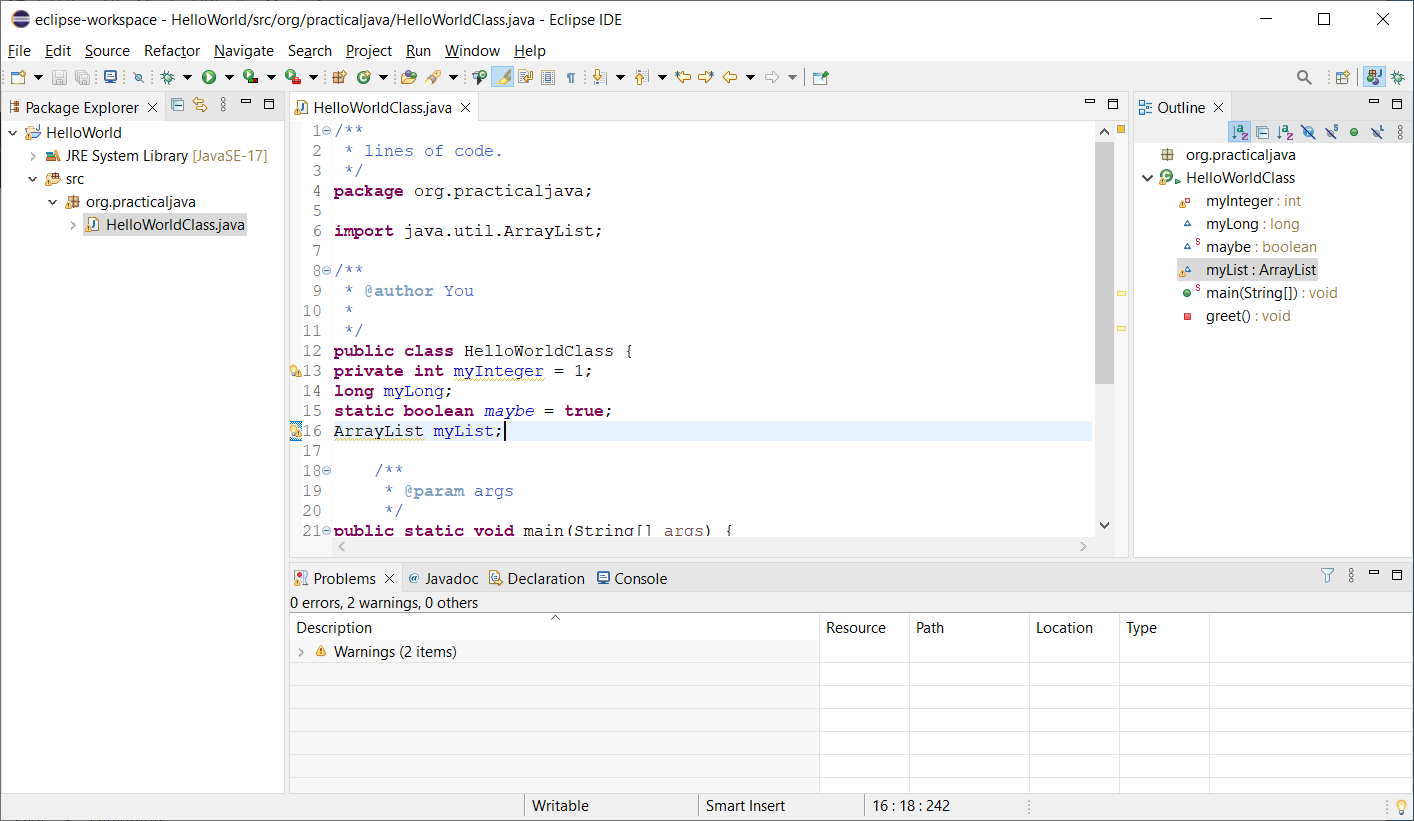
You can click on the Warnings leaf to expand it.
Formatting the Code
Finally, let’s format the code to make it easier to read. Press Ctrl+Shift+F (or menu item Source/Format) and see how things line up.
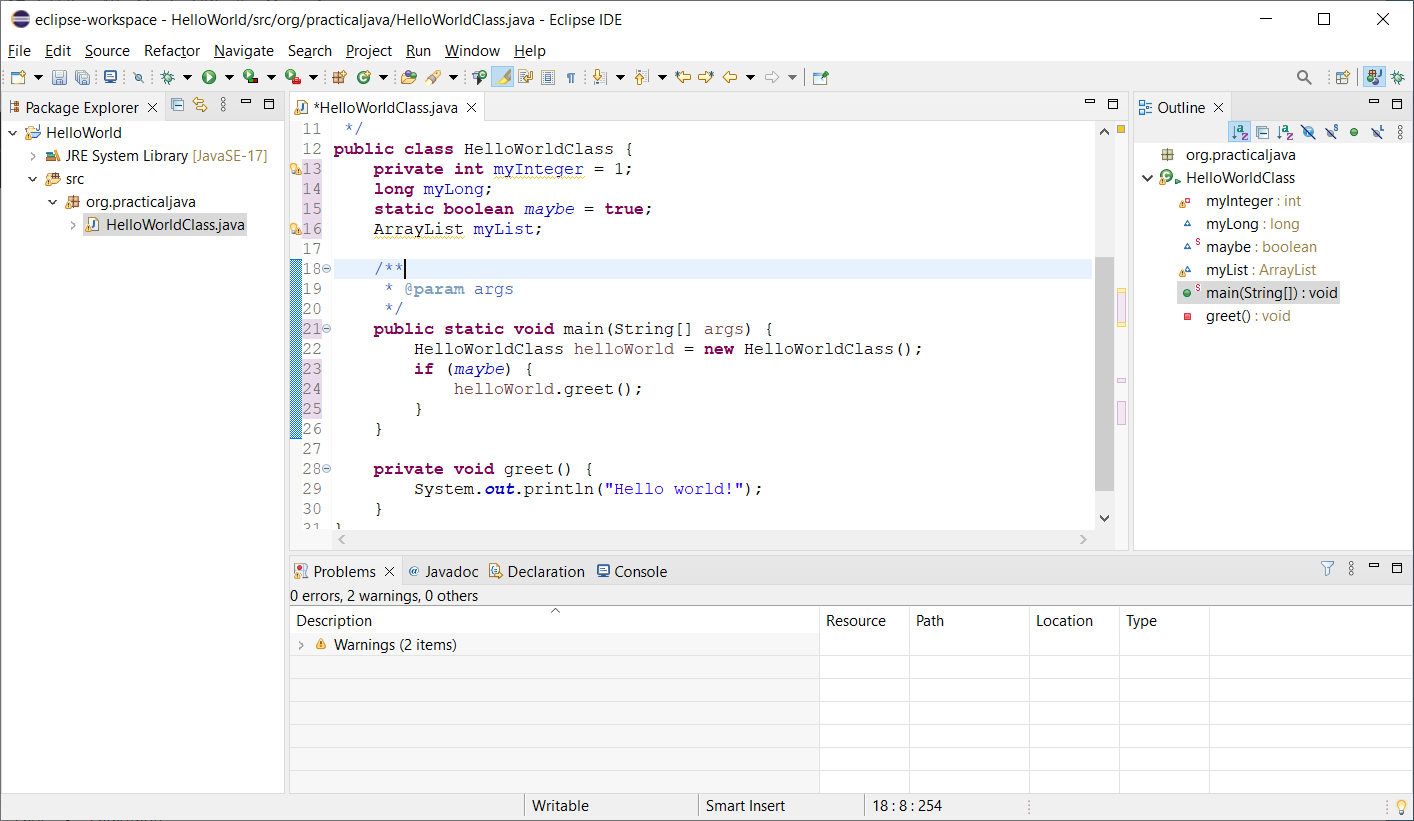
If you don’t select any code before reformatting, all the code in the class is affected. If you select lines first, only they are.
Let’s Run
For now, we’ll ignore the warnings. Let’s run our program. There are a few ways to do this:
- Right-click in the editor window and select Run As/Java Application from the popup menu.
- Right-click on the class file name in the Package Explorer and select Run As/Java Application from the popup menu.
- Select Run/Run (or, as before, Run As/Java Application) from the application menu.
You’ll be asked to save the HelloWorldClass.java if you haven’t already, and the program runs. Congratulations!
Previewing the command line
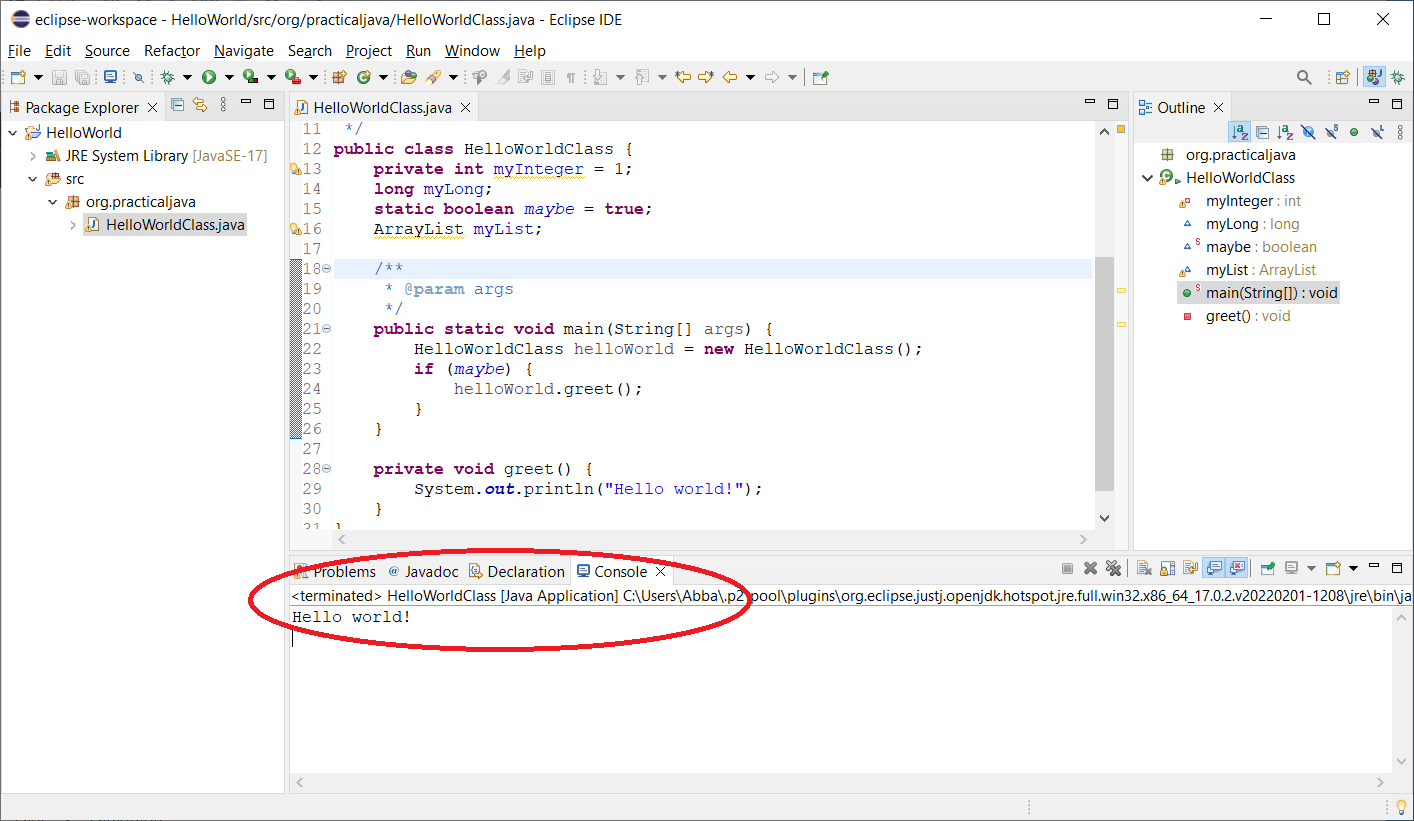
Note that the Console tab has moved to the front in the bottom window.
Refactoring
That name “maybe” is kind of odd, isn’t it? How about we change it? Like any good editor, the Eclipse Java editor has find and replace abilities. But the usual way of renaming is to use the Refactor/Rename command from the menu.
Start by putting the cursor anywhere within either occurrence of the name “maybe” and either selecting Refactor/Rename from the menu or pressing Shift+Alt+R. You’ll notice a rectangle appears around the name. Within the rectangle, replace “maybe” with “shouldPrint” and press Enter.
Did you notice that the other occurrence of the name changes in concert with your typing? It’s even better than that: when you refactor, any reference to the name throughout the project, including in other classes, changes too.
Refactoring includes other operations besides renaming, such as creating a constant from a literal, grouping statements within a method into a new method which can be called from elsewhere, and many others on the Refactor menu which we urge you to explore.
Finding a Declaration
Suppose the class you’re editing refers to something in another class, and you’d like a look at its definition. Just position the cursor within an occurrence of the name, and press F3. Try it by placing the cursor inside the name shouldPrint in “if (shouldPrint)” and pressing F3. The cursor moves to the definition of shouldPrint near the top. This also works if you hold down Ctrl and click on the item you’re interested in.
Of course, you’ll need this ability more when your class code is larger, or when the target item is in another class entirely. But trust me–you’ll be doing this a lot.
Compare and Replace
What happens when you screw up and want to back up to an earlier version of the file you’re editing? Eclipse has you covered. Right-click within the editor window, or on the file name in the Package Explorer, and choose Compare With or Replace With from the popup menu, and Local History from the sub-menu (or Previous from Local History from the Replace menu). If you choose Compare With/Local History, you’ll see a History window open, and if you double-click on one of the versions listed, you’ll see this:
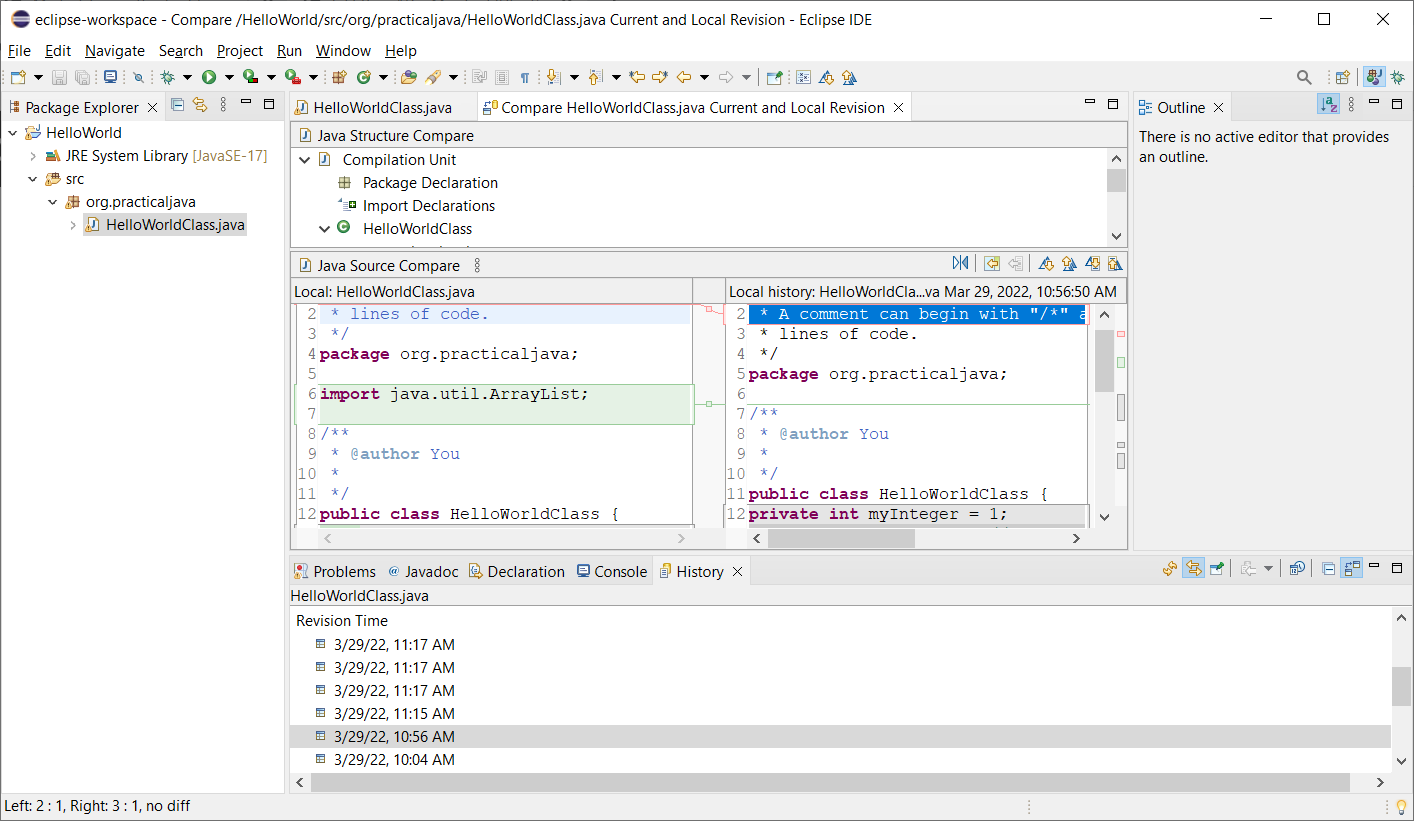
You can use the buttons at the right of the center window to jump from one difference to the next or previous, and copy lines from the older version to the current one. Replace works in similar fashion, but you can only replace the current version with the entire earlier one.
Click here to explore the Debugger.