A Java Tutorial Backed by Fifty Years of Experience
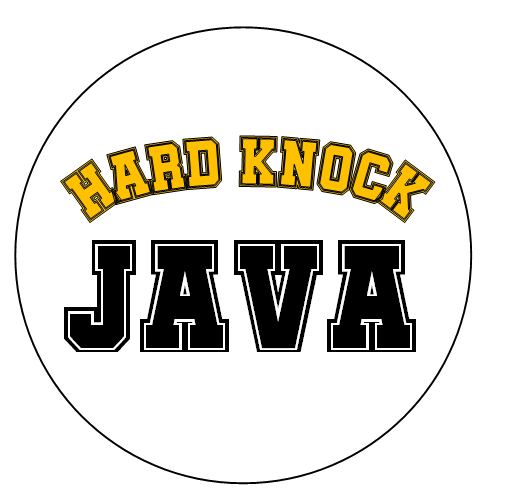
Welcome to Hard Knock Java, a free Java tutorial where you learn Java for free from a site whose name comes from its creator’s experience in the “school of hard knocks”: a nearly 50-year career in IT development.
Let’s Learn Java!
This is an introductory course in batch programming in the Java language. Along with some basic concepts, we aim to provide practical insights into the use of Java, and a brief look at some ways Java relates to the outside world: things like how to connect to a database, or get data from a remote source, for example.
Along the way we throw in anecdotes, quotes, observations on Java and on coding in general–plus elephant jokes, Burma Shave rhymes, one song parody, and one crossword puzzle. We’ve even got, via links, “guest lecturers” to elucidate the subject matter–but mostly to explain the pop culture references.
Getting the Most From This Java Tutorial
Since you’re reading this on a smart phone, we’ll suggest right now that you find yourself a laptop or desktop computer. To benefit from this course, you’ll need to establish a coding environment and run the examples and exercises–and you really don’t want to do that on a phone!
Remember the awkward feeling of getting behind the wheel of a car for the first time? And how with a little practice, you grew to be completely comfortable driving? A programming language is a lot like that: doing is the best way to learn. Just like in the classroom, we’ve got examples to study and exercises for you to try. Please don’t pass them up. As you work through them, your confidence builds until by the end you’re ready to solo.
Each of the exercises comes with a downloadable solution. But even if you don’t get stuck, it pays to compare your solution with mine. You may see something you hadn’t thought of. (This works both ways, of course. If you come up with a solution that’s more elegant than mine or more illustrative of the concepts being discussed, please let me know.)
It’s because of the examples and exercises that we advise you to take the course on a device with a dedicated keyboard rather than a phone.
Although you could pick up the course with any topic, it’s better to proceed in sequence. Some topics presume familiarity with earlier ones.
Wherever we introduce a standard Java class, you’ll find a link to its complete API (Application Programming Interface) documentation, which can give you a wealth of information about that class.
We also scatter other links throughout to videos and other pages just for fun and enlightenment. Enjoy!
A Brief Introduction to Java
So you may be thinking, why should I learn Java? Here are a few reasons.
First, Java is tremendously popular. It runs on all sorts of platforms, and is active on billions of devices–laptop and desktop computers (both PC and Mac), cell phones, microwave ovens, mainframes, you name it. It’s been around for roughly 25 years, so it’s got a solid track record.
Second, Java is device independent. Most programming languages behave differently on different CPUs or operating systems. For example, consider this data definition in COBOL:
77 MY-VARIABLE PIC S9(4) COMPUTATIONAL.
On the IBM System/360 platform, this is a signed 16-bit binary number between -32768 and 32767. On other platforms, it might be a four-digit decimal. But in Java, no matter where it runs, this:
int myNumber;
is ALWAYS a 32-bit signed binary number.
What makes this possible? The truth is, your compiled Java code doesn’t run directly on your phone, mainframe, or whatever. Instead, it runs on what we call a Java Virtual Machine (JVM for short). The JVM, a software component which is device specific, interprets the compiled Java code and makes it behave in a uniform manner, no matter where it was compiled or what it’s running on.
Third, Java is relatively easy to learn, compared to some other programming languages (especially C and C++), particularly with respect to memory management. Most modern computer languages allow you to allocate memory. As they do, they burden the developer the task of freeing it up, but Java doesn’t; once your program stops referring to something, it becomes eligible for garbage collection without you having to think about it.
Ready to get started? Great! We begin by setting up our learning environment. Click here to prepare.